It’s been a busy time for me. Mostly because I’m trying to learn new stuff, but also because I want to learn the new stuff in a right manner. In my previous post I started using WPF – Windows Presentation Foundation on a new interface. I used the designer and just created the GUI, and did not write a single line of code. I created the GUI based upon how I would do it in a Windows forms application.
Later on I started looking at how you do certain things in WPF, because the end goal was to actually learn something. Not do what I think is right and end up with spaghetti programming. After reading up on how to utilize WPF best I found that there’s a design pattern that is called MVVM. I have already encountered the MVC pattern and used the idea of it in a Unity application. Now before you faint, MVC in Unity was not the MVC as you find in web applications. It was called AMVCC, Application Model View Control Component. But the idea was mostly the same to separate the model from the view and have the controller do the work. The exception was that everything was run from the Application class, where everything resided (In a game object) and the hierchy inside Unity was Model, View, Control.
Confusious Confused Confusiado
Basically when starting out, what didn’t make sense to me was the word model (I was working in Unity at the time), was that model was the 3D models for me. However I have since learned that Model is the Data Model. Just thinking Data Model when thinking of Model made it so much clearer. View is simply the GUI, the GUI gets the data from the model. The control is the machine that works on the model and the model data is changed and reflected in the GUI. When I worked in Unity with AMVCC we used events to update data that the view then read and the data changed based on what the controller said the data was.
Therefore going to MVVM caused me a lot of head ache in WPF. I read pages upon pages about MVVM but it didn’t get any clearer. I contacted someone I know who just weeks before talked about MVVM and asked him and he said that he didn’t like it very much because it was a weird way of designing the app.
At this point I had a choice, I could make the app, the same way as I did with the windows forms version, ie in every button I would make a code snippet and open a form, pass the data I wanted back into the form and then make a very messy program. For a change I didn’t want to do what was easy, so I ended up taking an online course. I started looking at MVVM and found out that I need more knowledge. Not about MVVM firstly, but about how the WPF framework actually worked.
Back to the drawing board
I decided to take a step back and look at what makes WPF what it is. The answer is the underlying XAML. At first I thought I wouldn’t need to know anything about XAML to be able to make a WPF application. Turns out, that’s wrong.
I started with taking an online course in XAML, understanding what XAML is, what happens with it. Why you need x:Name on certain controls and why other controls used x:Key. Understanding how you nest the XAML statements and how binding works. Unfortunately I cannot teach the principles as well as the Pluralsight video did (they had two very brilliant videos on XAML and MVVM).
Anyway, what I didn’t understand (before I took the course) was that x:Name is how you can refer to the control. Let’s say you have a label, you want to access that, you can’t unless you give that label a name. Giving it a name let you access that control and change it’s content, color and whatnot. But doing that in code behind is sort of going against the whole MVVM pattern. But this is where it all fell into place. The XAML is the way you design the window, in the MVVM pattern you set up data binding to the ViewModel. The ViewModel is the mediator between the View and the Model.
It doesn’t stop there either, because Views can be both the GUI, but also a page. Pages are small GUI “snippets” that isn’t the complete view, but part of the GUI. As an example, I needed a color picker. But it doesn’t exist, so I downloaded the extended WPF toolkit, which contains the color picker as I wanted it, but it also contained a Color Canvas. In order to get the choice I wanted together I built a page where the page contained all of the things I was interested in. The page however is not a complete view, but rather something that pops up over the main window. But it is still a view.
The above is an example of a customized colorpicker. Basically the page pops up in a frame over the main window instead as a separate window. But in fact it is a separate window, which moves with the window attached inside.
MVVM – Commands – Databinding
XAML is a designer “language”. In Visual Studio there are tools to build the GUI. And the whole point with MVVM is to create a workflow where the GUI designer, design the GUI, using Visual Studio or Blend. The programmer then bind the different buttons to commands.
Turns out I needed to use another pattern called the Command Pattern. Command pattern is a simple pattern that basically issues commands and have a check to see if you can execute that command. In C# you have the Action and Predicate classes to use for that. Basically you inherit the ICommand interface and you have two methods. The Execute method and the CanExecute method.
Why is that important you may ask? The reason why this is important is that you use Commands on the buttons to perform the tasks you want. Here’s one example, this is how the color picker from above is displayed:
In XAML:
<Frame Source=”View/ColorSelectionView.xaml” Margin=”0,0,0,235″ NavigationUIVisibility=”Hidden” Visibility=”{Binding DisplayColorPicker, UpdateSourceTrigger=PropertyChanged}” Height=”424″ VerticalAlignment=”Center” Width=”259″ HorizontalContentAlignment=”Center” />
That means that the visibility is false at the start, because I set the ColorSelectionView Visibility to Visibility.Hidden. This is also important, it’s not a boolean, it is a Visibility.Hidden, Visibility.Visible and Visibility.Collapsed
To hide page again from the main view again XAML code:
<Grid HorizontalAlignment=”Right” VerticalAlignment=”Top”>
<i:Interaction.Triggers>
<i:EventTrigger EventName=”MouseDown”>
<i:InvokeCommandAction Command=”{Binding MainWindowVM.CloseColorDialog, UpdateSourceTrigger=PropertyChanged}”/>
</i:EventTrigger>
</i:Interaction.Triggers>
<Ellipse HorizontalAlignment=”Center” Height=”30″ Stroke=”#FF201A38″ VerticalAlignment=”Center” Width=”30″ Style=”{StaticResource CloseButtonElipseStyle}”/>
<TextBlock Foreground=”White” Text=”X” VerticalAlignment=”Center” HorizontalAlignment=”Center” FontSize=”20″/>
In the XAML code above, the visibility is bound to DisplayColorPicker through {Binding DisplayColorPicker}. DisplayColorPicker is a property in the ViewModel. I have made the mistake of having that in the MainViewModel, but the reason was that when I first started I wanted MainViewModel to invoke a completely new window. But I figued that why do that when you can display it over and disable the controls on the form.
Anyway apart from the mistake what you need is the following code:
private RelayCommand _openColorPicker;
private Visibility _colorPickerVisibility = Visibility.Hidden;
private bool _mainViewVisible = true; // Disble the buttons on the form
_openColorPicker = new RelayCommand(OpenColorPickerWindow, CanOpenColorWindow); // CanOpen can be null instead, chose to create the method because I wasn’t sure if I needed a CanOpenColorWindow.
private bool CanCloseColorPickerWindow(object obj)
{
return true;
}
private void CloseColorPickerWindow(object obj)
{
if (DisplayColorPicker == Visibility.Hidden)
{
DisplayColorPicker = Visibility.Visible;
DisplayMainViewContents = true;
}
else
{
DisplayColorPicker = Visibility.Visible;
DisplayMainViewContents = false;
}
}
public bool DisplayMainViewContents
{
get { return _mainViewVisible;}
set
{
_mainViewVisible = value;
OnPropertyChanged(“DisplayMainViewContents”);
}
}
public Visibility DisplayColorPicker
{
get
{
return _colorPickerVisibility;
}
set
{
if (_colorPickerVisibility == Visibility.Hidden)
{
_colorPickerVisibility = Visibility.Visible;
}
else
{
_colorPickerVisibility = Visibility.Hidden;
}
//OnPropertyChanged(“DisplayMainViewContents”);
OnPropertyChanged(“DisplayColorPicker”);
if (_colorPickerVisibility == Visibility.Visible)
{
_mainViewVisible = false;
}
else
{
_mainViewVisible = true;
}
OnPropertyChanged(“DisplayMainViewContents”);
}
}
public ICommand OpenColorDialog
{
get
{
if (_openColorPicker == null)
{
_openColorPicker = new RelayCommand(param=>this.OnOpenColorDialog());
}
return _openColorPicker;
}
}
public event EventHandler OpenColorDialogHandler;
void OnOpenColorDialog()
{
EventHandler handler = this.OpenColorDialogHandler;
if (handler != null)
{
handler(this, EventArgs.Empty);
}
}
In other words, a bucketload of code just to accomplish a small task. But the advantage is that once you know that you need to do all that, you can streamline the process. In my case, even thought the project is small, I was thinking of doing partial classes. Because as I have noticed thus far with C# is that the code gets large and it gets large fast, so the solution would be partial classes and then divide it up into fitting blocks. Like: MainWindowViewModel.ColorPicker ,
MainWindowViewModel.Ticker,
MainWindowViewModel.Image
etc.
I haven’t decided yet, but those parts are not related to each other, and since they aren’t the variables and methods can be on their own. Also I have in the past created a single method for Eventhandler like this:
private static void Handler(NoArgDelegate handler)
{
// ReSharper disable once UseNullPropagation – Doesn’t work in .net 2.0
if (handler != null) handler();
}
private static void Handler(SingleArgDelegate handler, object arg)
{
//Debug.Log(“Handled the event”);
if (handler != null && arg != null) handler(arg);
}
private static void Handler(DualArgDelegate handler, object arg1, object arg2)
{
if (handler != null && arg1 != null && arg2 != null) handler(arg1, arg2);
}
Then the handlers are used like this:
public static void FadeOutInterfaceTrigger()
{
Handler(OnFadeOutInterface);
}
public static void ReceivedGameImagePreset(object arg)
{
Handler(OnSetPresetGameImages, arg);
}
public static void ReceivedSpeedAndDuration(object arg1, object arg2)
{
Handler(OnReceiveSpeedAndDuration, arg1, arg2);
}
The drawback is that it can be error prone as you need to know the values coming in and cast them to their right type when getting the parameters.
Anyway that was a digression, moving back on track to the MVVM. The MVVM is a fairly complex pattern at first and it seems intimidating. The good news is that as long as you aren’t meddling with the code behind, there are some acceptable code you can use as code behind on the different views and that is code that is specific for that window. Close button behavior and what you click on to move the window (for instance). You can choose to do that with MVVM, but it is far easier to just add the proper events and do it that way.
What I didn’t get to above when it comes to Commands is that since events is done in the code behind, commands are what you use instead of events. Data binding you do to reflect data changing in the model or the view.
I’m going to end this with a Calvin and Hobbes strip. Because this is how it feels like working with MVVM at times. It’s when you question, what and why am I doing this and the obvious answer is right in front of you, because it’s a very good practice to do so, even if the code base gets much larger and more complex.
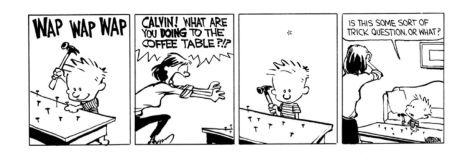